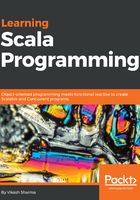
The for loop
In Scala, a for loop, also called for comprehension takes a sequence of elements, and performs an operation on each of them. One of the ways we can use them is:
scala> val stocks = List("APL", "GOOG", "JLR", "TESLA")
stocks: List[String] = List(APL, GOOG, JLR, TESLA)
scala> stocks.foreach(x => println(x))
APL
GOOG
JLR
TESLA
We defined a list named stocks with a few stock names. Then we used a simple for loop to print out each stock from that list. Take a look at the syntax: we have stock <- stocks that represents a single value from the list at the left-hand side of the generator sign <- and the list or sequence at the right-hand side. Then finally, we can provide any operation to be performed, in our case we printed the names. Now that we've seen how to write a simple for loop, let's define our set of printPages methods:
object PagePrinter extends App{
/*
* Prints pages page 1 to lastIndex for doc
*/
def printPages(doc: Document, lastIndex: Int) = if(lastIndex <= doc.numOfPages) for(i <- 1 to lastIndex) print(i)
/*
* Prints pages page startIndex to lastIndex for doc
*/
def printPages(doc: Document, startIndex: Int, lastIndex: Int) = if(lastIndex <= doc.numOfPages && startIndex > 0 && startIndex < lastIndex) for(i <- startIndex to lastIndex) print(i)
/*
* Prints pages with given Indexes for doc
*/
def printPages(doc: Document, indexes: Int*) = for(index <- indexes if index <= doc.numOfPages && index > -1) print(index)
/*
* Prints pages
*/
private def print(index: Int) = println(s"Printing Page $index.")
println("---------Method V1-----------")
printPages(Document(15, "DOCX"), 5)
println("---------Method V2-----------")
printPages(Document(15, "DOCX"), 2, 5)
println("---------Method V3-----------")
printPages(Document(15, "DOCX"), 2, 5, 7, 15)
}
/*
* Declares a Document type with two arguments numOfPages, typeOfDoc
*/
case class Document(numOfPages: Int, typeOfDoc: String)
The following is the output:
---------Method V1-----------
Printing Page 1.
Printing Page 2.
Printing Page 3.
Printing Page 4.
Printing Page 5.
---------Method V2-----------
Printing Page 2.
Printing Page 3.
Printing Page 4.
Printing Page 5.
---------Method V3-----------
Printing Page 2.
Printing Page 5.
Printing Page 7.
Printing Page 15.
We have a utility print method, which does nothing but print a simple string with index numbers, you're free to imagine a real printer printing the pages though.
Our definition for printPages method version 1 merely consists of a condition check that the document consists of the pages to be printed. That's done via an if conditional statement. More on if statements later in this chapter. After the conditional statement, there's a loop on indexes which ranges from 1 to the lastIndex passed. The same way the other method version 2 is also defined that takes startIndex and lastIndex and prints pages for you. For the last method version 3 of printPages, we're looping on the indexes passed and we have a condition guard that starts with an if statement. This checks whether the page index is less than the number of pages in the document passed as an argument, and prints it. Finally, we got the result expected from our methods.