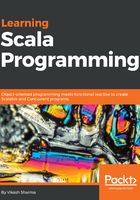
Looping
Standing in front of a printer, you give it an instruction to print pages with indexes 2 to 16 from your book. The printer, which is programmed to do so, uses an algorithm to print pages for you; it checks for the document and the number of pages you asked to print. It sets the starting point as 2 and the last point as 16, and it starts printing till the last point is reached. Printing pages we can call repetitive, thus printing each page from your document can be well programmed using a looping construct. As in any other language, Scala supports for, while, and do while loops.
Take a look at the following program:
object PagePrinter extends App {
/*
* Prints pages page 1 to lastIndex for doc
*/
def printPages(doc: Document, lastIndex: Int) = ??? //Yet to be defined
/*
* Prints pages page startIndex to lastIndex for doc
*/
def printPages(doc: Document, startIndex: Int, lastIndex: Int) = ???
/*
* Prints pages with given Indexes for doc
*/
def printPages(doc: Document, indexes: Int*) = ???
/*
* Prints pages
*/
private def print(index: Int) = println(s"Printing Page $index.")
}
/*
* Declares a Document type with two arguments numOfPages, typeOfDoc
*/
case class Document(numOfPages: Int, typeOfDoc: String)
We have created an object named PagePrinter. We use the syntax /* ... */ to declare multi-line comments and // for single line comments in Scala. We declared three methods, which are supposed to do what the comment says. These methods are yet to be defined and we have communicated that to the Scala compiler, how? Using the syntax "???", that is, three question mark symbols, we tell the Scala compiler we're yet to define the method.
Let's come back to our methods. The first one takes a document, the number of pages to print as arguments, and prints pages up to the passed index. The second one takes the start and end indexes of pages to print and does so. The third method can take random indexes to print and prints pages from those indexes. In the third method, we've used an asterisk * to make our Int argument a vararg, that is, a variable argument. Now, the task is to define these methods. We can also see that to define what a document is, we have used a case class—we'll learn about case classes when we go deeper into Scala's object-oriented part in the next few chapters. For now, it'll be helpful to know that a case class let's you create a class with all the boilerplate code already available for you; it means you can access the members, in our case, numOfPages and typeOfDoc. Well, there's a lot to know about case classes, but we'll go through it later. We'll use our looping constructs to define our PagePrinter.
Let's take a look at our looping constructs. We'll first go through the for loop.