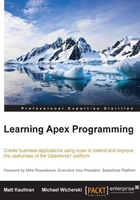
Exceptions prove the rule
Like other programming languages, Apex includes a built-in class for exceptions. When your code exceeds a limit, an exception is thrown. For this reason, you really need to understand how exceptions work in Apex before we can go any further on limits. When the Salesforce1 Platform throws an exception, all statements that have been executed within that transaction are rolled back causing your entire attempt to execute code to fail. It's not fun to get an exception; it means that an end user is upset and you have more work to do.
Apex includes a generic exception class as well as over 20 types of specific exceptions for when various rules are broken. A lot of these specific exception types are easy to avoid such as don't divide by 0 when doing math, don't refer an index of a list that is out of bounds, and don't construct an account and then add it to a list of contacts. You get the picture. When your code does cause one of these exceptions, you can usually avoid it with an if
statement. The following screenshot displays the exception types included in Apex, but you can learn more from Force.com Apex Code Developer's Guide at http://developer.salesforce.com:

If you like to play it safe, you can take advantage of Apex's exception handling abilities. You can use a try-catch
block around your code as an insurance policy against exceptions. This will allow your code to continue to run after the exception is thrown by the Salesforce1 Platform so that you can handle the error gracefully. This is shown in the following code snippet:
//Try breaking a rule Integer x = 10; try { x = x/0; } catch ( MathException e){ system.debug('Go back to school!'); } finally { system.debug(x); }
The previous code block demonstrates how to use a try-catch
block with the optional finally
statement.
The most common exception type is the dreaded NullPointerException
. It is thrown anytime you reference an object as if it wasn't null, but it really is. This happens anytime you write code expecting a certain situation, but instead the unexpected occurs. It doesn't matter whether your business requirements dictate that a value should exist, you always need to write your code to avoid this exception. This is shown in the following code
//Here's what not to do Integer x; Integer y = 5+x; //You can't divide by null //Do this instead If ( x != null ){ y = 5+x; } String z; System.debug(z.length()); //You can't call a method on null //Do this instead If ( z != null ){ System.debug(z.length()); } List<String> stringList; System.debug(stringList[0]); //You can't reference a nonexistent object //Do this instead if ( stringList != null && stringList.size() > 0 ){ system.debug(stringList[0]); } Boolean myFlag; if ( myFlag == true ) { system.debug('This Boolean isn't set yet'); } //Do this instead if ( myFlag != null && myFlag == true ){ system.debug('Wow, you read this book'); }
This example demonstrates just some of the extremely common situations that lead to NullPointerException
and how you can easily avoid them with the use of a single if
statement. It's only one additional line of code to avoid NullPointerException
, that's a great deal!