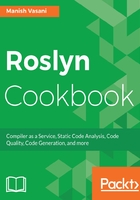
How it works...
Syntax node analyzers register one or more syntax node action callbacks to analyse syntax kinds of interest. We specified interest in analyzing VariableDeclaration syntax kind in the RegisterSyntaxNodeAction invocation.
context.RegisterSyntaxNodeAction(syntaxNodeContext =>
{
...
}, SyntaxKind.VariableDeclaration);
Analysis works by operating on the syntax node and semantic model exposed off the syntax node analysis context in the callback. We first do syntactic checks to verify that we are operating on a valid implicitly typed declaration:
// Do not flag implicitly typed declarations that declare more than one variables,
// as the compiler already generates error CS0819 for those cases.
var declaration = (VariableDeclarationSyntax)syntaxNodeContext.Node;
if (!declaration.Type.IsVar || declaration.Variables.Count != 1)
{
return;
}
We then perform semantic checks using the semantic model APIs to get semantic type information about the type declaration syntax node and verify it is not an error type or primitive system type:
// Do not flag variable declarations with error type or special System types, such as int, char, string, and so on.
var typeInfo = syntaxNodeContext.SemanticModel.GetTypeInfo(declaration.Type, syntaxNodeContext.CancellationToken);
if (typeInfo.Type.TypeKind == TypeKind.Error || typeInfo.Type.SpecialType != SpecialType.None)
{
return;
}
If both the syntactic and semantics check succeed, then we report a diagnostic about recommending explicit type instead of var.