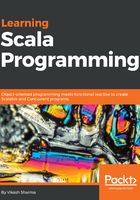
The for expressions
We've seen the for loops, and how simple it is to use them in Scala. There's much more we can do with the for syntax. Here's an example:
object ForExpressions extends App {
val person1 = Person("Albert", 21, 'm')
val person2 = Person("Bob", 25, 'm')
val person3 = Person("Cyril", 19, 'f')
val persons = List(person1, person2, person3)
for {
person <- persons
age = person.age
name = person.name
if age > 20 && name.startsWith("A")
} {
println(s"Hey ${name} You've won a free Gift Hamper.")
}
case class Person(name: String, age: Int, gender: Char)
}
The following is the result:
Hey Albert You've won a free Gift Hamper.
In the preceding example, we used a generator, definitions, and filters in the for expression. We used a for expression on a list of persons. We proposed a gift hamper for a person whose name starts with A and who is older than 20 years of age.
The first expression in for is a generator expression which generates a new person from the persons list and assigns to person. Second is age and name definitions. Then finally we apply filters using the if statement to put conditions for our winner:

What if we want a couple more prizes for our people. In that case we may want to get a sub list of winners. That's possible by introducing yield.