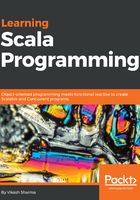
Data types
We have just covered literals in Scala, and with that, we've almost covered the introduction to all the data types existing as well. We discussed how to define Int, Long, Short, and Byte data types. Along with these, we also covered Float and Double type. Together, all these are called numeric data types. The Byte, Short, and Char are called sub-range types. We also talked about Boolean, character, and strings:

In Java, these numeric types are called Primitive Types, and then there are user-defined types as well. But in Scala, these somewhat similar types to primitives, are called value types. Objects of these value types are not represented by an object in the underlying runtime system. Thus, arithmetic operations performed are in the form of methods defined for Int, and other numeric value types. Think about it, it means that we can perform method operations on these. So let's take an example:
scala> val x = 10 //x is an object of Type Int
x: Int = 10 //x is assigned value 10
scala> val y = 16 //y is an object of Type Int
y: Int = 16 //y is assigned value 16
scala> val z = x + y //z is addition of x and y's value
z: Int = 26
As you can see, x and y are two Integer objects created, and there's another named z. The result is z, addition of x and y. The sign + here is a method on the Int object, which means more than just an operator, it's a method that is defined for Int types and expects a parameter of Int type. This is going to have a definition similar :
scala> def +(x: Int): Int = ??? //Some definition
$plus: (x: Int)Int
What do we get from this? It means that the construct is more powerful because the methods look more natural and can also be written for other types. And that's how it's written for Int. Let's try this:
scala> val aCharAndAnInt = 12 + 'a'
aCharAndAnInt: Int = 109
This happened because there's a method + overloaded for the type character. Something like this:
scala> def +(x: Char): Int = ???
$plus: (x: Char)Int
You may refer to the class Int.scala at http://www.scala-lang.org/api/2.12.0/scala/Int.html, and go through how these methods are structured. I would recommend taking a closer look at the source of this class, and see if there's anything particular.