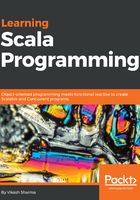
Tuple literals
Tuple is a data type in Scala. We'll discuss the type in this chapter later on. First, let's take a look at how we can define a literal of the same type:
scala> val aTuple = ("Val1", "Val2", "Val3")
aTuple: (String, String, String) = (Val1,Val2,Val3)
scala> println("Value1 is: " + aTuple._1)
Value1 is: Val1
Here, we defined a Tuple3, which took three parameters that are in parentheses and comma separated. Its type is going to be Tuple3, the same way we can define TupleN with N being 1 to 22. Take a closer look at the REPL response for the first declaration:
aTuple: (String, String, String) = (Val1,Val2,Val3)
Here aTuple has a Type (String, String, String), so as we assigned values to our identifier, aTuple Scala was able to construct type based on values we gave. Tuple values can be accessed using a special underscore syntax. Here, we use the tuple attribute name, along with an underscore (_), followed by the index of value. In our example, we're using val1, so we gave aTuple._1 value.
A tuple with two elements is also called a Pair, it can be defined using the arrow assoc (->) operator:
scala> val smartPair = 1 -> "One"
smartPair: (Int, String) = (1,One)