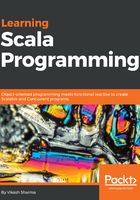
Integer literals
Numeric literals can be expressed in the form of decimal, octal, or hexadecimal forms. These are basic integer values that can be signed or unsigned. Octal values are deprecated since version 2.10, so if you try out a numeric with a leading 0, it'll give you a compile time error:
scala> val num = 002
<console>:1: error: Decimal integer literals may not have a leading zero. (Octal syntax is obsolete.)
val num = 002
^
If you define a literal with prefix 0x or 0X, it's going to be a hexadecimal literal. Also, the Scala interpreter prints these values as a decimal value. For example:
scala> 0xFF
res0: Int = 255
The value to be printed is converted to its decimal equivalent, and then printed. Hexadecimal literals can contain digits (0 to 9) and letters (A to F) in upper/lower case. Integer literals are further classified into different types such as Int, Long, Byte, and Short literals. These literals are divided based on the range of values. The following table shows the minimum and maximum values for specified types:

If we try to define any literal outside of these ranges for specified types, the compiler is going to give some error stating type mismatch:
scala> val aByte: Byte = 12
aByte: Byte = 12
Here, we defined a normal Byte value with type information given explicitly. If we try to give a value that is out of the range for a Byte, the compiler will try to convert that value to an integer, and then try to assign it to the attribute, but will fail to do so:
scala> val aByte: Byte = 123456
<console>:20: error: type mismatch;
found : Int(123456)
required: Byte
val aByte: Byte = 123456
This happened because the compiler tries to assign the converted value of Int(123456) to aByte, which is of Byte type. Hence, the types do not match. If we do not use type explicitly, then Scala itself is capable of inferring the type due to type inference. What if we try to assign an attribute, an integer value that does not come under any of the mentioned ranges? Let's try:
scala> val outOfRange = 123456789101112131415
<console>:1: error: integer number too large
val outOfRange = 123456789101112131415
In these cases, the Scala compiler is smart enough to sense that things are out of control, and gives this error message stating integer number too large.
To define long literals, we put the character L or l at the end of our literal. Otherwise, we can also give type information for our attribute:
scala> val aLong = 909L
aLong: Long = 909
scala> val aLong = 909l
aLong: Long = 909
scala> val anotherLong: Long = 1
anotherLong: Long = 1
The Byte and Short values can be defined by explicitly telling the interpreter about the type:
scala> val aByte : Byte = 1
aByte: Byte = 1
scala> val aShort : Short = 1
aShort: Short = 1