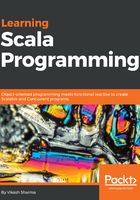
What is underneath a Scala program?
A Scala program is a tree of nested definitions. A definition may start with a keyword, definition's name, a classifier, and if it's a concrete definition, then also an entity to which that definition is bound. So the syntax is regular, just like any other programming language has keyword/name/classifier/bound-entity. Let's take an example. We'll use Scala REPL to see how a simple Scala program is built. For that, let's import a Scala package named universe:
scala> import scala.reflect.runtime.universe._
import scala.reflect.runtime.universe._
This import clause brings all the definitions within the universe package in scope. It means that the required functions we are going to use are in scope, and available for us to use. Then we'll use a reify method, which returns an Expr to construct tree out of our simple Scala program expression. We passed a Scala class to our reify method. Let's pretend that a Scala class encapsulates some members like a value with the name segment and a name definitions. We'll talk about all these members as we go along in subsequent sections. For now, let's execute this line and see what we get in response:
scala> val expr = reify {class Car {val segment="SUV"; def name="Q7"}}
expr: reflect.runtime.universe.Expr[Unit] =
Expr[Unit]({
class Car extends AnyRef {
def <init>() = {
super.<init>();
()
};
val segment = "SUV";
def name = "Q7"
};
()
})
The preceding code shows Scala's reify method's response. It looks like alien code (only for now) that we have no idea about, so let's find out what's meaningful to us. We know which is somewhat using the class Car that we passed to generate some code. We recognize this Car class and that it's extending some construct named AnyRef. Every class we define in Scala is a subclass of AnyRef, hence we can see the interpreter has shown the explicit view of our class definition with modifiers, constructors, and members that we defined. We'll use the showRaw(expr.tree) method to print tree:
scala> showRaw(expr.tree)
res0: String = Block(List(ClassDef(Modifiers(), TypeName("Car"), List(), Template(List(Ident(TypeName("AnyRef"))), noSelfType, List(DefDef(Modifiers(), termNames.CONSTRUCTOR, List(), List(List()), TypeTree(), Block(List(Apply(Select(Super(This(typeNames.EMPTY), typeNames.EMPTY), termNames.CONSTRUCTOR), List())), Literal(Constant(())))), ValDef(Modifiers(), TermName("segment"), TypeTree(), Literal(Constant("SUV"))), DefDef(Modifiers(), TermName("name"), List(), List(), TypeTree(), Literal(Constant("Q7"))))))), Literal(Constant(())))
Now, we'll take a closer look at the response res0. The expression started with Block, that's a tree representing the class we defined. Our class Car contained a value declaration named segment, and a method named name. The representation of our class as a tree contains all the entities that we defined. And these together construct our program. A tree that we got using the method showRaw(tree) give us the skeleton of the program we wrote. The tree has String literals like SUV and Q7, value definitions like segment, and other meaningful constructs. We'll learn the basics of these literals and types of data in Scala in this chapter.