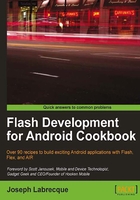
Detecting whether or not a device supports multitouch
When developing projects which target the Android operating system, it is always a good idea to make sure that multitouch is actually supported on the device. In the case of an Android phone, this will probably always be the case, but what about a Google TV or AIR for TV device? Many of these are also Android-based yet most televisions do not have any touch control whatsoever. Never assume the capabilities of any device.
How to do it...
We will need to use internal classes to detect whether or not multitouch is supported:
- First, import the following classes into your project:
import flash.display.StageScaleMode; import flash.display.StageAlign; import flash.display.Stage; import flash.display.Sprite; import flash.text.TextField; import flash.text.TextFormat; import flash.ui.Multitouch;
- Declare a
TextField
andTextFormat
object to allow visible output upon the device:private var traceField:TextField; private var traceFormat:TextFormat;
- We will now set up our
TextField
, apply aTextFormat
, and add it to theDisplayList
. Here, we create a method to perform all of these actions for us:protected function setupTextField():void { traceFormat = new TextFormat(); traceFormat.bold = true; traceFormat.font = "_sans"; traceFormat.size = 44; traceFormat.align = "center"; traceFormat.color = 0x333333; traceField = new TextField(); traceField.defaultTextFormat = traceFormat; traceField.selectable = false; traceField.mouseEnabled = false; traceField.width = stage.stageWidth; traceField.height = stage.stageHeight; addChild(traceField); }
- Then, simply invoke
Multitouch.supportsGestureEvents
andMultitouch.supportsTouchEvents
to check each of these capabilities as demonstrated in the following method:protected function checkMultitouch():void { traceField.appendText(String("Gestures: " + Multitouch.supportsGestureEvents) + "\n"); traceField.appendText(String("Touch: " + Multitouch.supportsTouchEvents)); }
- Each of these properties will return a
Boolean
value oftrue
orfalse
, indicating device support as shown here:
How it works...
Detecting whether the device supports either touch or gesture events will determine how much freedom you, as a developer, have in refining the user experience. If either of these items returns as false, then it is up to you to provide (if possible) an alternative way for the user to interact with the application. This is normally done through Mouse
events: